Turn APIs into SDKs that developers love
Turning APIs into developer-friendly SDKs:
2930 SDKs*based on the total npm downloads
and counting...
Shopify GraphQL Storefront API
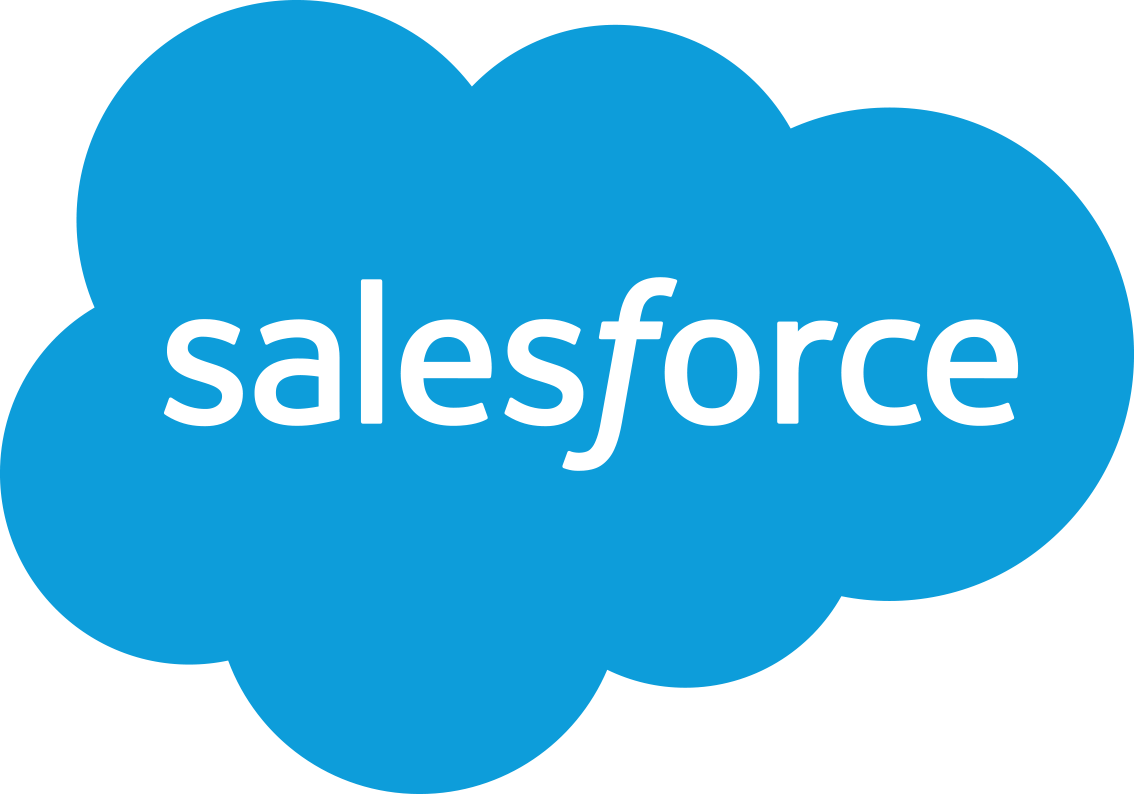
Salesforce GraphQL Developer API
Contentful.com GraphQL API
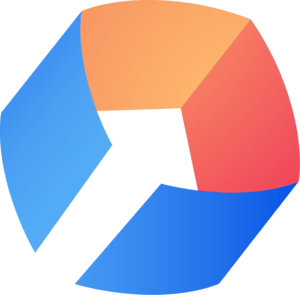
Stigg.com GraphQL Server-API
Fully featured SDKs in seconds.
Ergonomic shortcuts for common API patterns, that feel natural.
No need to look up API docs or write boilerplate code.
Typesafety & True Custom Scalars
Forget about type casting, manual type checking or deserializing scalar values.
It's all there, out of the box.
Immediate Type Safety
(1)Immediate Type Safety
Changes reflect immediately
(2)Changes reflect immediately
While typing your queries with full type and autocompletion support, your return type is immediately inferred.
No need to manually define types or run additional code generation steps.
import spacex from "spacex";const company = await spacex.query.company(({ceo, // Stringfounded, // Int}) => ({ceo,founded,}));console.log(company);const founded = company.founded;const foundedIn2002 = founded === 2002;// ✅ Type OK// ✅ (founded is a number)// { ceo: 'Elon Musk', founded: 2002 }
Custom Scalars
Custom scalars have to be serialized for network transport and often remain a string in most GraphQL clients , leaving you with the burden of deserializing them manually.
Not anymore. Dates and JSONs are automatically (lazily) deserialized for you and you can define your own custom scalars with full typesafety.
Full support for Custom Scalars
(1)Full support for Custom Scalars
Custom scalars have to be serialized for network transport and often remain a string in most GraphQL clients, leaving you with the burden of deserializing them manually.
Not anymore. Dates and JSONs are automatically (lazily) deserialized for you and you can define your own custom scalars with full typesafety.
import sdk from "sdk";const timeslots = await sdk.query.availableSlots(({start, // DateTimeend, // DateTimeisBooked, // Boolean}) => ({start,end,isBooked,}));console.log(timeslots.filter((s) => !slot.isBooked).filter((s) => {return s.start >= new Date()});// ----------- 👆 no need to parse,// it's already a Date object!);
Custom Scalars with custom parse
(1)Custom Scalars with custom parse
Custom Scalars with custom types
(2)Custom Scalars with custom types
You can define your own custom scalars with full type safety.
For example, you can define a custom scalar for a DateTime
scalar type and convert a Unix timestamp to a Date
object.
import sdk from "sdk";sdk.init({scalars: {DateTime: (v: string) => {return new Date(+v * 1000)},// ---- 👆 typesafe function:// (v: string) => Date// for example,// convert a Unix timestamp// to a Date object},});const timeslots = await sdk.query.availableSlots(({start, // DateTime}) => ({start,}));console.log(timeslots.filter((s) => {return s.start >= new Date()});// ---------- 👆 no need to parse, it's already a Date object!);
Immediate Type Safety
While typing your queries with full type and autocompletion support, your return type is immediately inferred.
No need to manually define types or run additional code generation steps.
Changes reflect immediately
If you change the query, the type of the result will reflect the changes immediately.
For example, if you change the founded
field to be an alias for the ceo
field, the type will change accordingly and you will get a type error.
Full support for Custom Scalars
Custom scalars have to be serialized for network transport and often remain a string in most GraphQL clients, leaving you with the burden of deserializing them manually.
Not anymore. Dates and JSONs are automatically (lazily) deserialized for you and you can define your own custom scalars with full typesafety.
Custom Scalars with custom parse
You can define your own custom scalars with full type safety.
For example, you can define a custom scalar for a DateTime
scalar type and convert a Unix timestamp to a Date
object.
Custom Scalars with custom types
You can even use your own classes or types for custom scalars.
The function you define is typesafe and will be used to deserialize the scalar value, so all fields of that type will be automatically deserialized with that function and be of the correct type.
import spacex from "spacex";const company = await spacex.query.company(({name,ceo,founded, //}) => ({name,ceo,founded, //}));// typeof company: {// name: string,// ceo: string,// founded: number //// }console.log(company);const foundedIn2002 = company.founded === 2002;// ✅ Type OK (founded is a number)// { name: 'SpaceX', ceo: 'Elon Musk', founded: 2002 }
Authentication
Authenticate one or all requests with ease.
No weird hacks or workarounds. Just a simple, clean API.
Without Auth
(1)Without Auth
.auth("token")
(2).auth("token")
.auth({ Authorization: "token" })
(3).auth({ Authorization: "token" })
.auth( async () => "token" | { Authorization: "token" })
(4).auth( async () => "token" | { Authorization: "token" })
Globally with .init({ auth: ... })
(5)Globally with .init({ auth: ... })
If no global authentication is set, the SDK will send requests without any authentication headers.
import sdk from "sdk";const me = await sdk.query.me(({ id, username, email }) => ({id,username,email,}));// ❌ Error! 401 Unauthenticatedconsole.log(me);